AWS EC2에 Redis 생성 & Spring boot 연동법
- 웹 개발
- 2023. 10. 1.
EC2 우분투 22.04 버전으로 설치 된 가정하에 작성한다
우분투 apt-get을 업그레이드 해준다.
sudo apt-get update
sudo apt-get upgrade
Redis 설치를 해주자.
sudo apt-get install redis-server
# 설치확인
redis-server --version
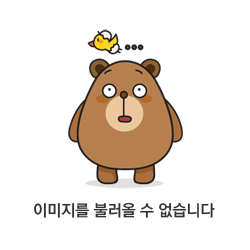
이렇게 나오면 성공이다.
이제 Redis를 외부접속이 가능하게 설정해주자.
sudo vi /etc/redis/redis.conf
/bind
# 입력후 n 누르면 계속 찾기가 됨.
# i 누르면 입력으로 전환 됨
# 127.0.0.1 ::1 -> 0.0.0.0 ::1 바꾸기
# 바꿧다면 esc 누른다
/foobared
# 입력후 n 누르면 계속 찾기가 됨.
# requirepass foobared 에서 foobared 부분을 원하는 비밀번호로 바꿔주고 #을 없애준다
# 메모리 max 값 설정하기
/maxmemory
# 입력후 n 누르면 계속 찾기가 됨.
# maxmemory <bytes> 에서 # 제거 후 <bytes> 부분에 500mb라고 적자
# 프리티어 기준으로 1gb밖에 되지 않아 500mb로 설정해줬다
# 메모리 교체 알고리즘 변경
/maxmemory-policy
# 입력후 n 누르면 계속 찾기가 됨.
# maxmemory-policy noeviction 에서 # 제거 후 noeviction 부분을 allkeys-lru로 바꿔주자.
# 다 바꿧으면 esc 누르고 :wq! 입력하면 나오게 된다
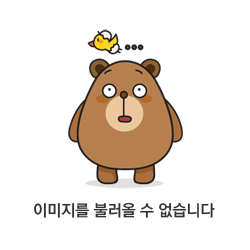
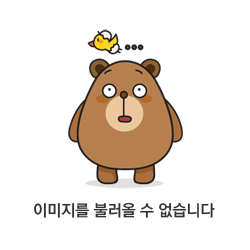
메모리 교체 알고리즘은 위와 같이 필요한거 사용하면 된다.
밑으로 내리다보면
bind 127.0.0.1 ::1 이렇게 있을거다 이것을 bind 0.0.0.0 ::1 으로 바꿔준다.
i 누르면 수정 상태로 바뀌고
수정이 되었다면 esc 누른다음 :wq! 입력하면 된다.
이제 한번 접속을 해보자.
redis는 인 메모리 데이터베이스이기 때문에 실행중인 상태여야 접근이 가능하다
sudo systemctl start redis-server
이제 보안그룹에서 인바운드 규칙을 설정해줘야 한다
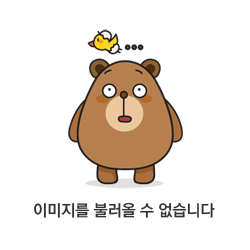
Redis 기본포트인 6379를 열어줘야 한다
이제 ec2 말고 로컬 컴퓨터로 접속해보자
로컬에 redis 설치가 되어 있어야 한다.
redis-cli -h {ec2 아이피} -p 6379 -a {설정한 비밀번호}
입력하면
아이피:포트> 이렇게 뜨면서 완료가 된다.
Spring boot 설정
build.gradle
implementation 'org.springframework.boot:spring-boot-starter-data-redis'
application.properties
spring.redis.host=localhost
spring.redis.port=6379
spring.redis.password=비밀번호
RedisConfig.class
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.connection.lettuce.LettuceConnectionFactory;
import org.springframework.data.redis.core.RedisTemplate;
@Configuration
public class RedisConfig {
@Value("${spring.redis.host}")
private String host;
@Value("${spring.redis.port}")
private int port;
@Value("${spring.redis.port}")
private String password;
@Bean
public RedisConnectionFactory redisConnectionFactory() {
RedisStandaloneConfiguration configuration = new RedisStandaloneConfiguration();
configuration.setHostName(host);
configuration.setPort(port);
configuration.setPassword(password);
return new LettuceConnectionFactory(configuration);
return new LettuceConnectionFactory();
}
@Bean
public RedisTemplate<String, Object> redisTemplate() {
RedisTemplate<String, Object> redisTemplate = new RedisTemplate<>();
redisTemplate.setConnectionFactory(redisConnectionFactory());
return redisTemplate;
}
}
Entity RefreshToken.class
package project1.OurFit.entity;
import lombok.Getter;
import org.springframework.data.annotation.Id;
import org.springframework.data.redis.core.RedisHash;
import org.springframework.data.redis.core.index.Indexed;
@Getter
@RedisHash(value = "refreshToken", timeToLive = 1209600) // 2주
public class RefreshToken {
@Id // Redis Key 값이 되며 null로 세팅하면 랜덤값으로 설정
@Indexed // 이 값으로 데이터를 찾을 수 있게 설정 (findByEmail 처럼)
private String email;
private String refreshToken;
public RefreshToken(String email, String refreshToken) {
this.email = email;
this.refreshToken = refreshToken;
}
}
RefreshTokenRedisRepository.class
import org.springframework.data.repository.CrudRepository;
import project1.OurFit.entity.RefreshToken;
import java.util.Optional;
public interface RefreshTokenRedisRepository extends CrudRepository<RefreshToken, String> {
Optional<RefreshToken> findByEmail(String email);
}
환경을 다 만들어줬으니 사용하면 된다